This post shows you how you can handle Boolean
types in an Angular
application using Pipe
. The Pipe
formerly known as Filter
in AngularJs
is an Angular feature that helps transform data. Example shows a very simple pipe that converts a boolean
value to a formatted string (yes or no).
Create a pipe without any hassle with the Angular CLI tool using the following command,
ng g pipe yesNo
import { Pipe, PipeTransform } from "@angular/core";
@Pipe({
name: "yesNo"
})
export class YesNoPipe implements PipeTransform {
transform(value: any, ...args: any[]): any {
return value ? "Yes" : "No";
}
}
Make the yesNo
pipe globally available so that you can use it in any component you like:
import { BrowserModule } from "@angular/platform-browser";
import { NgModule } from "@angular/core";
import { ReactiveFormsModule } from "@angular/forms";
import { AppRoutingModule } from "./app-routing.module";
import { AppComponent } from "./app.component";
import { PointsTableComponent } from "./points-table/points-table.component";
import { YesNoPipe } from "./shared/yes-no.pipe";
@NgModule({
declarations: [AppComponent, PointsTableComponent, YesNoPipe],
imports: [BrowserModule, ReactiveFormsModule, AppRoutingModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
Use the pipe like the following while interpolation:
<div class="columns">
<div class="column is-half">
<table class="table is-fullwidth is-hoverable is-striped">
<thead>
<tr>
<th><abbr title="Team Name">Team</abbr></th>
<th><abbr title="Played">Pld</abbr></th>
<th><abbr title="Won">W</abbr></th>
<th><abbr title="Lost">L</abbr></th>
<th><abbr title="Points">Pts</abbr></th>
<th>Qualified for Semi Finals</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let item of data">
<td>{{ item.team }}</td>
<td>{{ item.played }}</td>
<td>{{ item.won }}</td>
<td>{{ item.lost }}</td>
<td>{{ item.points }}</td>
<td
[ngStyle]="{
color: item.isQualified
? 'hsl(141, 53%, 53%)'
: 'hsl(348, 100%, 61%)'
}"
>
<b>{{ item.isQualified | yesNo }}</b>
</td>
</tr>
</tbody>
</table>
</div>
<div class="column">
<pre class="user-pre">{{ structure | json }}</pre>
</div>
</div>
Here, data
is just a json
array field on your component. Here goes the full script of the component.
import { Component, OnInit } from "@angular/core";
import {
FormGroup,
FormControl,
FormBuilder,
Validators
} from "@angular/forms";
@Component({
selector: "app-points-table",
templateUrl: "./points-table.component.html",
styleUrls: ["./points-table.component.scss"]
})
export class PointsTableComponent implements OnInit {
structure: any;
data: Array<any>;
ngOnInit() {
this.structure = {
team: "string",
played: "number",
won: "number",
lost: "number",
points: "number",
isQualified: "boolean"
};
this.data = [
{
team: "AUS",
played: 9,
won: 7,
lost: 2,
points: 14,
isQualified: true
},
{
team: "ENG",
played: 9,
won: 6,
lost: 3,
points: 12,
isQualified: true
},
{
team: "NZ",
played: 9,
won: 5,
lost: 3,
points: 11,
isQualified: true
},
{
team: "SA",
played: 9,
won: 3,
lost: 5,
points: 7,
isQualified: false
},
{
team: "WI",
played: 9,
won: 2,
lost: 6,
points: 5,
isQualified: false
}
];
}
}
Repository:
https://github.com/fiyazbinhasan/ng-playground/tree/ng-playground-pipes
Links:
https://angular.io/guide/pipes
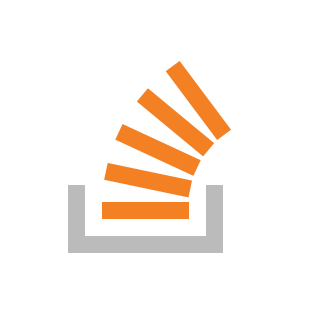
Comments